Legend State
High performance state and local first sync
Legend State is an extremely fast signal-based state library with fine-grained reactivity and a powerful sync engine that works with any backend.
const speed$ = observable(2) const Component = () => { // Get and observe it const speed = use$(speed$) // Set it const up = () => speed$.set(v => v % 10 + 1) return (<> {/* Two way bind it */} <$React.input $value={speed$} type="number" /> <Button onClick={up}>{speed} is too slow 🤘</Button> </>) }
All in One
Legend-State is the fastest React state library, and it takes care of all of the hard sync and caching stuff for you.
⚡️ Fine-grained reactivity in React
Achieve incredible performance by minimizing the number and size of renders.
Legend State makes apps fast by default because they just do less work.
Normal React
Legend-State
🦄 Incredibly easy to use
When you get() values while observing, it tracks them and re-runs when they change. No boilerplate, no selectors, no dependency arrays, just easy reactivity.
const settings$ = observable({ ui: { theme: 'dark' }}) // Infinitely nested observables const theme$ = settings$.ui.theme // get returns the raw data theme$.get() // 'dark' // set sets theme$.set('light') // Computed observables with just a function const isDark$ = observable(() => theme$.get() === 'dark' ) // observe re-runs when observables change observe(() => { console.log(theme$.get()) }) // use$ re-renders when observables change const Component = () => { const theme = use$(settings$.ui.theme) return <div>{theme}</div> })
🚀 The fastest React state library
Legend-State is so fast that it even outperforms vanilla JS in some benchmarks. It's extremely optimized with fine-grained reactivity and massively reduces re-rendering.
🤝 Local State = Remote State
Just get and set observables and they sync themselves with a powerful sync engine. Your UI code doesn't need any querying, creating mutations, or synchronizing with local state.
You can even bind UI components directly to synced observables.
You don't need any sync code in your components. You can just focus on making great apps.
const profile$ = observable(syncedFetch({ get: 'https://myurl/my-profile', set: 'https://myurl/my-profile', persist: { plugin: ObservablePersistLocalStorage, name: 'profile', }, })) const Component = () => { // get triggers fetch and updates on change const name = use$(profile$.name) const onClick = () => { // set sets the local state and syncs profile$.name.set('Annyong') } // Two-way bind to remote data return <$React.input $value={profile$.name} /> }
Local First with any backend
Use one of our ever-expanding library of sync plugins or build your own on top of the CRUD plugin or the basic synced plugin.
Backend
Persistence
const { mutations, queries } = client.api const messages$ = observable(syncedKeel({ list: queries.listMessages, create: mutations.createMessages, update: mutations.updateMessages, delete: mutations.deleteMessages, // Persist locally persist: { plugin: ObservablePersistLocalStorage, name: 'messages', retrySync: true // Retry sync after reload }, changesSince: 'last-sync' // Sync only diffs }))
Legend Kit
Both an expertly crafted starter kit and an intelligent coding assistant, it's the perfect starting point to build great apps even faster.
📚 Tons of tools to get started quickly
High performance headless components, general purpose observables, transformer computeds, React hooks that don't re-render, and observable tools for popular frameworks.
Optimized Components
- Tabs
- Modals
- Forms
- Toasts
- More...
Observable helpers
- currentDate
- createDraft
- stringAsNumber
- setAsString
- More...
React hooks
- useMeasure
- usePosition
- useScrolled
- useHover
- More...
Framework hooks
- useRoutes
- useRouteHistory
- useCanRender
- usePauseProvider
- More...
🧑💻 VS Code Extension
A contextually aware coding assistant to accelerate your development speed
Snippets
Easily add Legend-State features by hotkey
Smart Generation
Quickly generate full components
Auto Imports
Automatically adds imports as needed
Context-Aware Sidebar
Quick access to tools most useful in any moment
Linter
Detect and fix common issues like missing observer
Extensive Customization
Customize to your workflow and create your own snippets
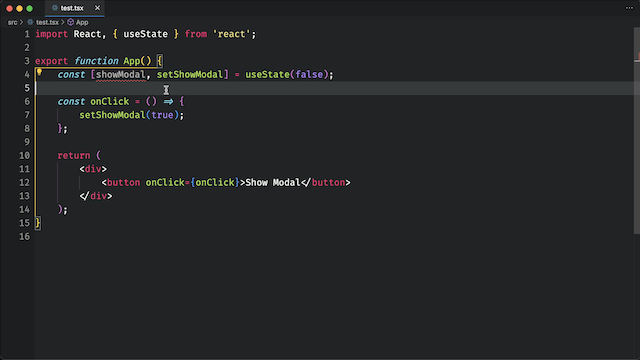
🤗 Reactive Components for your favorite UI kit
Augment your UI kit with reactive props and two-way binding
👩🏫 Example projects
Full open-source apps built with Legend-State that you can use as a starting point or for reference for best practices.
Buy once, yours forever
Lifetime access to an ever-growing library of helpers, components, hooks, example projects, and reactive components.
Now available for pre-order at a discount for a limited time.
Releasing shortly after Legend-State 3.0.