Introduction
Legend-State is a super fast all-in-one state and sync library that lets you write less code to make faster apps. Legend-State has four primary goals:
1. 🦄 As easy as possible to use
There is no boilerplate and there are no contexts, actions, reducers, dispatchers, sagas, thunks, or epics. You can structure your data however you want in local or global stores. It doesn’t modify your data at all, and you can just call get()
to get the raw data and set()
to change it.
import { observable, observe } from "@legendapp/state"import { observer } from "@legendapp/state/react"
const settings$ = observable({ theme: 'dark' })
// get returns the raw datasettings$.theme.get() // 'dark'// set setssettings$.theme.set('light')
// Computed observables with just a functionconst isDark$ = observable(() => settings$.theme.get() === 'dark')
// observing contexts re-run when tracked observables changeobserve(() => { console.log(settings$.theme.get())})
const Component = observer(function Component() { const theme = state$.settings.theme.get()
return <div>Theme: {theme}</div>})
React components track access to get()
on observables and automatically re-render whenever they change.
import { observable } from "@legendapp/state" import { observer } from "@legendapp/state/react" // Create an observable object const settings$ = observable({ theme: 'dark' }) // This is the code for the example on your right -----> const Component = observer(function Component() { // theme is automatically tracked for changes const theme = settings$.theme.get() const toggle = () => { settings$.theme.set(theme => theme === 'dark' ? 'light' : 'dark' ) } return ( <Box theme={theme}> <div>Theme: {theme}</div> <Button theme={theme} onClick={toggle}> Toggle theme </Button> </Box> ) })
2. ⚡️ The fastest React state library
Legend-State beats every other state library on just about every metric and is so optimized for arrays that it even beats vanilla JS in some benchmarks. At only 4kb
and with the massive reduction in boilerplate code, you’ll have big savings in file size too.
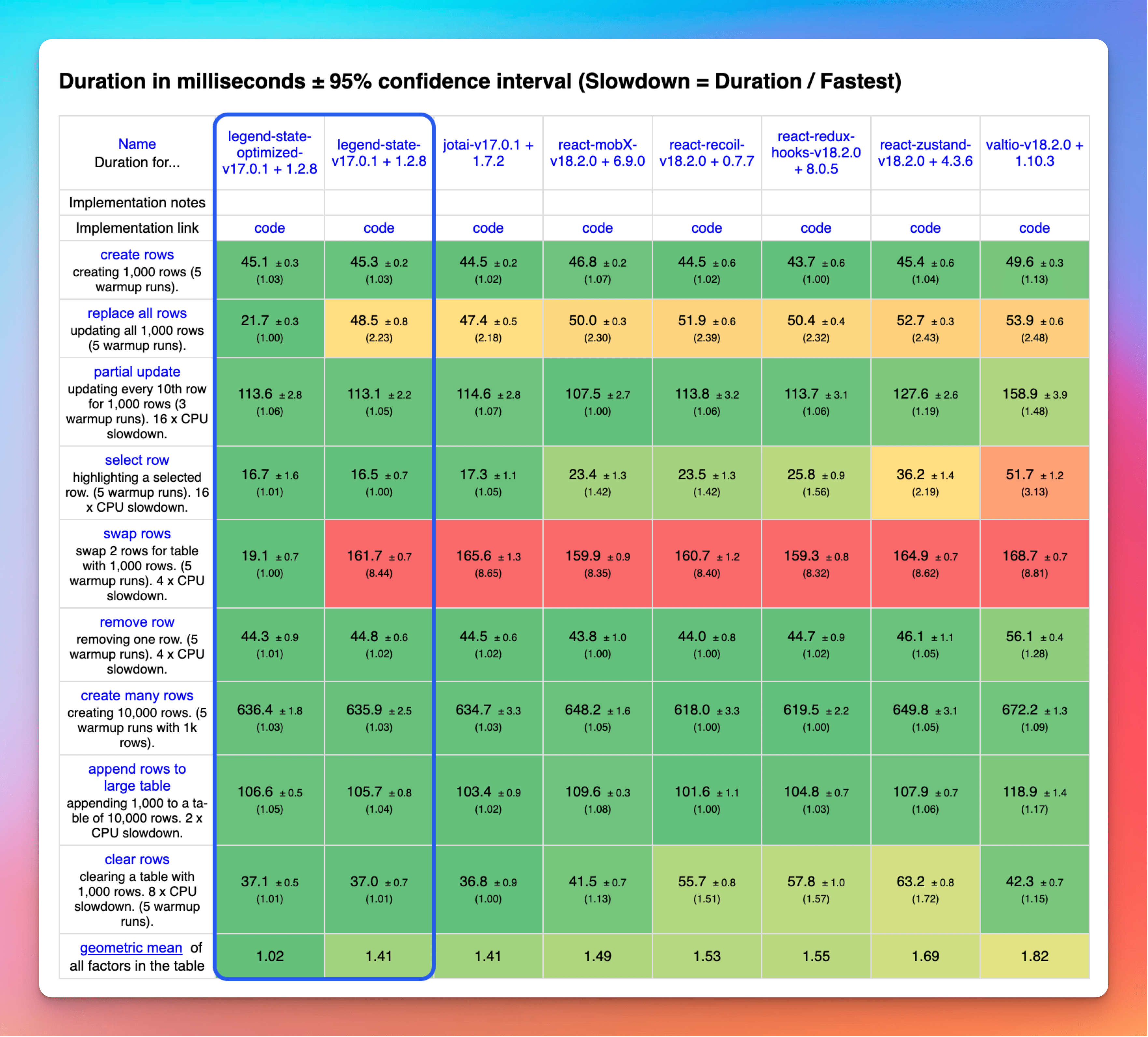
See Fast 🔥 for more details of why Legend-State is so fast.
3. 🔥 Fine-grained reactivity for minimal renders
Legend-State helps your components re-render less, less often, making your apps faster 🔥.
import { observable } from "@legendapp/state" import { Memo, useObservable } from "@legendapp/state/react" import { useRef, useState } from "react" import { useInterval } from "usehooks-ts" function NormalComponent() { const [count, setCount] = useState(1) const renderCount = useRef(1).current++ useInterval(() => { setCount((v) => v + 1) }, 600) // This re-renders when count changes return ( <FlashingDiv> <h5>Normal</h5> <div>Renders: {renderCount}</div> <div>Count: {count}</div> </FlashingDiv> ) } function FineGrained() { const count$ = useObservable(1) const renderCount = useRef(1).current++ useInterval(() => { count$.set((v) => v + 1) }, 600) // The text updates itself so the component doesn't re-render return ( <FlashingDiv> <h5>Fine-grained</h5> <div>Renders: {renderCount}</div> <div>Count: <Memo>{count$}</Memo></div> </FlashingDiv> ) }
4. 💾 Powerful sync and persistence
Legend-State includes a powerful sync and persistence system. It easily enables local-first apps by optimistically applying all changes locally first, retrying changes even after restart until they eventually sync, and syncing minimal diffs. We use Legend-State as the sync systems in Legend and Bravely, so it is by necessity very full featured while being simple to set up.
Local persistence plugins for the browser and React Native are included, with sync plugins for Keel, Supabase, TanStack Query, and fetch
.
const state$ = observable( users: syncedKeel({ list: queries.getUsers, create: mutations.createUsers, update: mutations.updateUsers, delete: mutations.deleteUsers, persist: { name: 'users', retrySync: true }, debounceSet: 500, retry: { infinite: true, }, changesSince: 'last-sync', }), // direct link to my user within the users observable me: () => state$.users['myuid'])
observe(() => { // get() activates through to state$.users and starts syncing. // it updates itself and re-runs observers when name changes const name = me$.name.get()})
// Setting a value goes through to state$.users and saves update to serverme$.name.set('Annyong')
Install
Version 3 is currently available in the @alpha version and may change before the final release.
Highlights
- ✨ Super easy to use 😌
- ✨ Super fast ⚡️
- ✨ Super small at 4kb 🐥
- ✨ Fine-grained reactivity 🔥
- ✨ Built-in sync system
- ✨ No boilerplate
- ✨ Designed for maximum performance and scalability
- ✨ React components re-render only on changes
- ✨ Very strongly typed with TypeScript
- ✨ Persistence plugins for automatically saving/loading from storage
- ✨ State can be global or within components
The core is platform agnostic so you can use it in vanilla JS or any framework to create and listen to observables. It includes support for React and React Native, and has plugins for automatically persisting to storage.
Read more about why you’ll love Legend-State ❤️
Getting Started
Continue on to Getting Started to get started!
Community
Join us on Discord or Github to get involved with the Legend community.
Contributing
We welcome contributions! Please read our Contributing Guide on Github